The blog post introduces an important data type, Maybe, in Haskell.
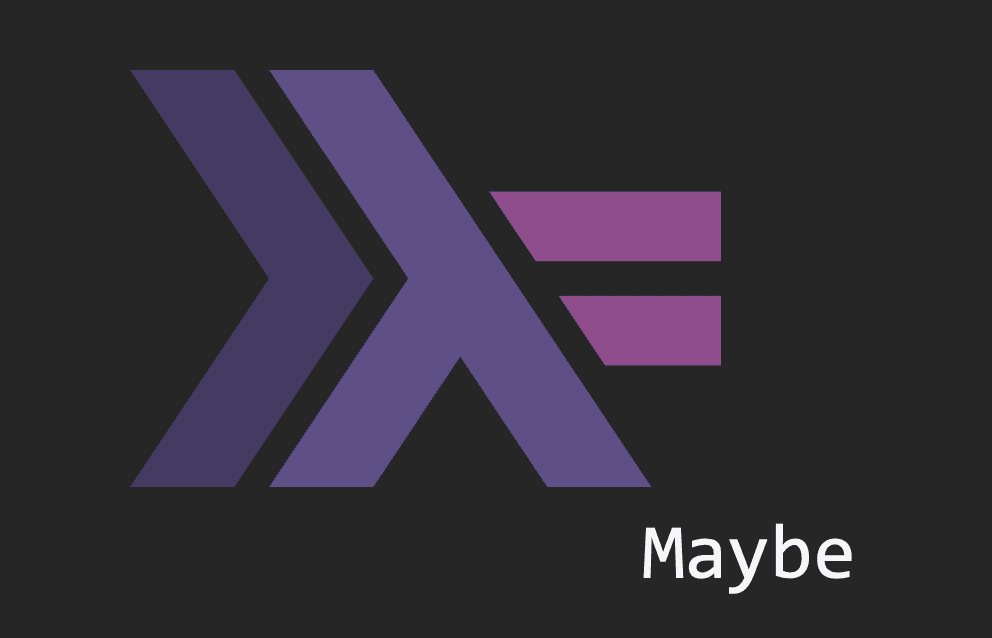
Maybe
If you have experience coding in different languages, you must have encountered numerous errors and exceptions and wondered how they can be
handled in Haskell. In Haskell, we use the Maybe
datatype.
data Maybe = Nothing | Just a
The datatype can be used as follows:
safediv :: Integral a => a -> a -> Maybe a
safediv a b =
if b == 0 then Nothing else Just $ div a b
We can see from the above that it allows us to safeguard against the special case where division would throw an error -
when the denominator is zero. This will result in the following behavior from safediv
:
safediv 10 2
=> Just 5
safediv 10 0
=> Nothing
Excellent.
Usage
As we use the Maybe
datatype quite notoriously in Haskell, there are useful predefined functions available.
import Data.Maybe
isJust :: Maybe a -> Bool
isNothing :: Maybe a -> Bool
fromJust :: Just a -> a
We can use fromJust
to extract a value in the Just
constructor, but applying this to Nothing
accidentally will result in an error.
Hence, we can use fromMaybe
from Data.Maybe
, which can be defined as follows:
fromMaybe :: a -> Maybe a -> a
fromMaybe d x
| isNothing x = d
| otherwise = fromJust x
This function provides a default value (d) in case the Maybe
value is Nothing
, ensuring safer extraction of values. We also have another
useful function called maybe
.
maybe :: b (a -> b) Maybe a b
maybe d f x
| isNothing x = d
| otherwise = f x
This is similar to how fromMaybe
works, but it allows us to apply function on Maybe
only when Maybe
is Just
.
Exercises
This is an exercise section where you can test your understanding of the material introduced in the article. I highly recommend solving these questions by yourself after reading the main part of the article. You can click on each question to see its answer.
Resources
- Philipp, Hagenlocher. 2020. Haskell for Imperative Programmers #14 - Maybe. YouTube.