The blog post introduces a very useful datatype, Either, in Haskell.
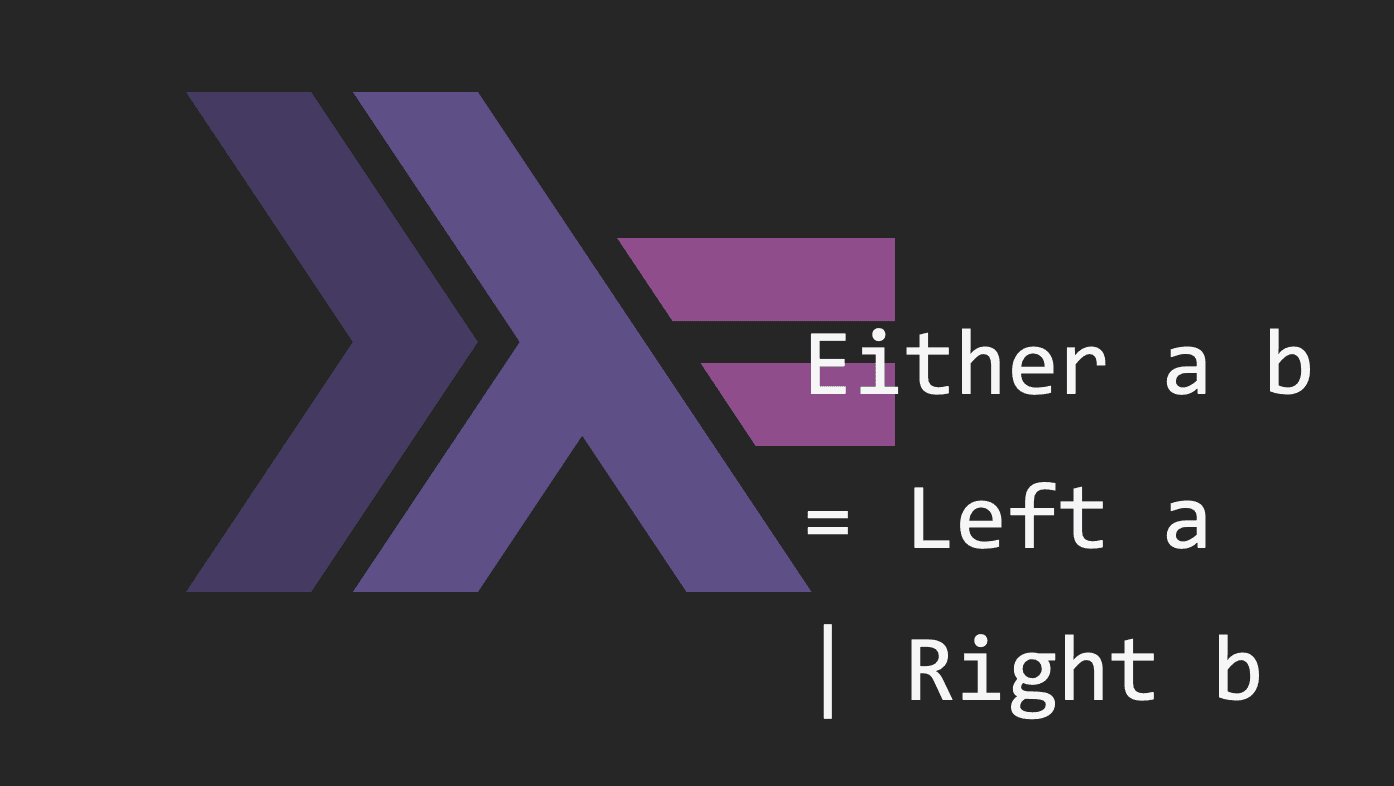
Either
There are many situations where you want to have some data that can either be Int
or String
, or
any pairs of data types. In such cases, the Either
datatype can be quite helpful.
data Either a b = Left a | Right b
Using Either
, we can construct something like the example below.
data Either a b = Left a | Right b
type someData = Either Int String
[Left 1, Right "Hello"] :: [someData]
Predefined Functions
Because Either
is such a helpful datatype, Haskell has prepared some predefined some functions for Either
.
import Data.Either
-- Extract Lefts
lefts :: [Either a b] -> [a]
-- Extract Rights
rights :: [Either a b] -> [b]
-- Return if Either is Left
isLeft :: Either a b -> Bool
-- Return if Either is Right
isRight :: Either a b -> Bool
The above functions are trivial, but there are two interesting functions with Either
, which are either
and
partitionEither
.
-- Apply function corresponding to datatype in Either
either :: (a -> c) -> (b -> c) -> Either a b -> c
-- Partition the values of Lefts and Rights in a tuple of lists
partitionEither :: [Either a b] -> ([a], [b])
Using either
we can do something like the example below.
f = either (\l -> "Err: Number is provided.") (\r -> "Hello, "+r+"!") -- partial function application
The above example hints that Either
can be used for error handling, similar to Maybe
. The difference is that
while Nothing
holds no value, Left
can preserve the value inside, which can be useful when you want to
display the details of the error. In the next article, we will see how Either
can be helpful when throwing
an exception.
Exercises
This is an exercise section where you can test your understanding of the material introduced in the article. I highly recommend solving these questions by yourself after reading the main part of the article. You can click on each question to see its answer.
Resources
- Philipp, Hagenlocher. 2020. Haskell for Imperative Programmers #22 - Either. YouTube.